Facebook api login example
Summary
I want that my WebApp authenticate the user with facebook. Once it is done, the WebApp will send the facebookToken to the api, and thanks to that the api will be able to have access to facebook user infomation. And we will be able to create a JsonWebToken to authenticate our REST api.
You can find the source code in github : https://github.com/Tkanos/FacebookRestAuthentication
Code
You can find the source code in github : https://github.com/Tkanos/FacebookRestAuthentication
So we need to have an web app that connect on facebook and receive the facebooktoken.
In our API, we will need to have a Login endpoint to receive this facebooktoken , sent by the web app.
var getToken = function(req, res) {
var facebookToken = req.headers['facebooktoken'];
//TODO : check the expirationdate of facebooktoken
if(facebookToken) {
var path = 'https://graph.facebook.com/me?access_token=' + facebookToken;
var path = 'https://graph.facebook.com/me?access_token=' + facebookToken;
request(path, function (error, response, body) {
var facebookUserData = JSON.parse(body);
var facebookUserData = JSON.parse(body);
if (!error && response && response.statusCode && response.statusCode == 200) {
if(facebookUserData && facebookUserData.id) {
var accessToken = jsonWebToken.sign(facebookUserData, jwtSecret, {
//Set the expiration
expiresIn: 86400
});
//Set the expiration
expiresIn: 86400
});
res.status(200).send(accessToken);
} else {
res.status(403);
res.send('Access Forbidden');
}
res.status(403);
res.send('Access Forbidden');
}
}
else {
console.log(facebookUserData.error);
//console.log(response);
res.status(500);
res.send('Access Forbidden');
}
});
res.status(403);
res.send('Access Forbidden');
}
};
else {
console.log(facebookUserData.error);
//console.log(response);
res.status(500);
res.send('Access Forbidden');
}
});
res.status(403);
res.send('Access Forbidden');
}
};
As you can see receiving the facebookToken we get all the information the user share with us calling :
'https://graph.facebook.com/me?access_token=' + facebookToken;
Once we have the answer, that is all information of our user on Json. We can “Sign” it on jsonwebtoken (eventually save it in our database). and send back our new token that will be use to communicate with our api.
Be careful, for security purpose sign a jsonwebtoken is not enough, you will also need to encrypt it. On this example we only sign, but in your own code don’t forget to encrypt.
As you can see on API/apiRouter.js (see on github), we will get the jsonwebtoken on every communication, we have “verified” it in order to have our user information back in json.
apiRouter.use('/', function(req, res, next){
var jwt = req.headers.authorization;
if(jwt) {
var jwtManager = require('./Login/loginController')();
jwtManager.verify(accessToken, function(err, decoded){
if(err) {
res.status(401).send('Invalid Token');
}
else {
req.connectedUser = decoded;
next();
}
});
}
else {
next();
}
});
So in all methods of my application I will potentially have the userconnected attached to the request.
req.connectedUser
How to test this Api
To test we will need to have a facebook Token. You can get one for test on https://developers.facebook.com/tools/explorer/?method=GET&path=me&version=v2.7

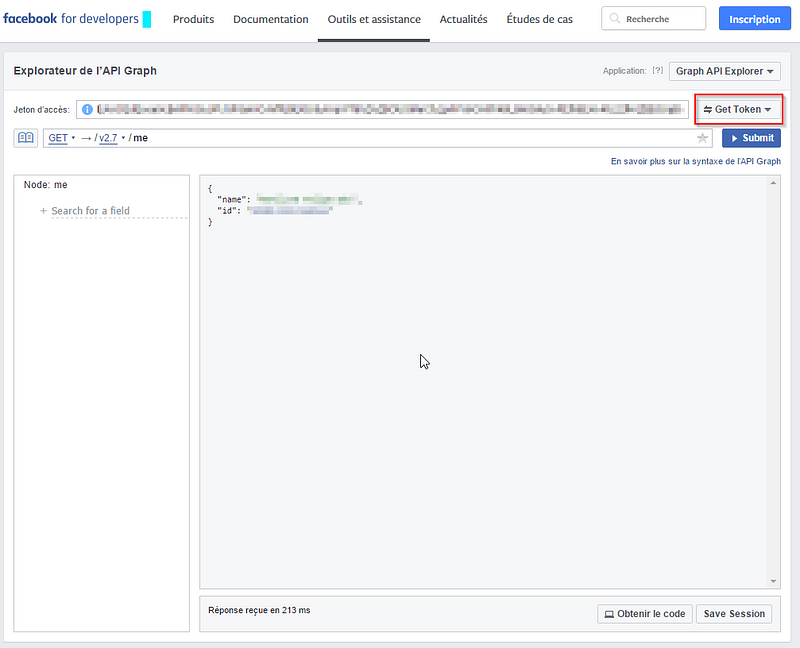
Then (after have done a npm start), you can use a REST CALL to our Login service. put your facebooktoken on the header, you will receive the jsonwebtoken on the response:

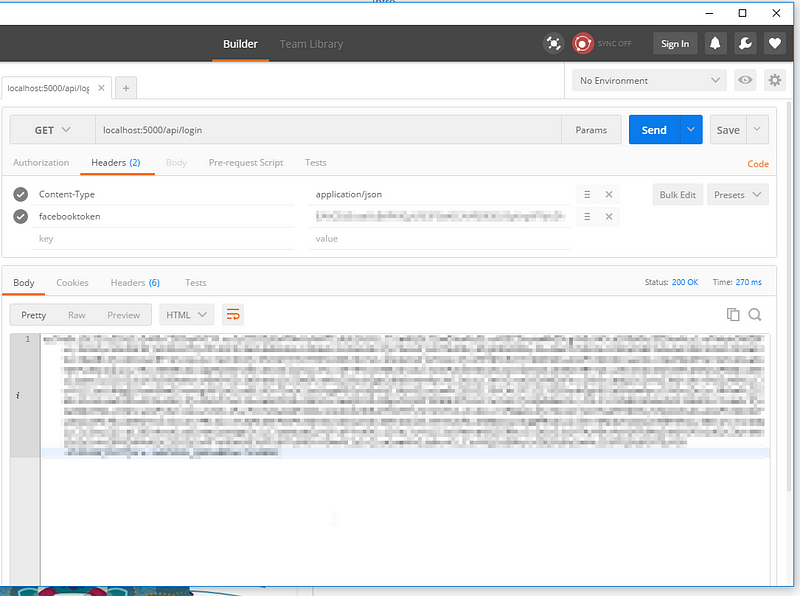
Link
Facebook api login example
Reviewed by Daniel Chuks
on
03:47
Rating:
No comments